Custom form with a separate page creation is similar to a page creation described here. First thing we obviously need a custom module. Let's use the simple module created here. Next step is to define our form page using snippets.routing.yml:
snippets.custom_form:
path: '/custom-form'
defaults:
_form: '\Drupal\snippets\Form\CustomForm'
_title: 'Custom form page'
requirements:
_permission: 'access content'
Ther difference here is that we use _form instead of _controller. The Next and the last step is to create form class in snippets/src/Form/CustomForm.php:
<?php
namespace Drupal\snippets\Form;
use Drupal\Core\Form\FormBase;
use Drupal\Core\Form\FormStateInterface;
/**
* Simple form with first and last names to enter.
*
* @internal
*/
class CustomForm extends FormBase {
/**
* {@inheritdoc}
*/
public function getFormId() {
return 'custom_form';
}
/**
* {@inheritdoc}
*
* @param array $form
* A nested array form elements comprising the form.
* @param \Drupal\Core\Form\FormStateInterface $form_state
* The current state of the form.
*/
public function buildForm(array $form, FormStateInterface $form_state) {
$form['first_name'] = [
'#type' => 'textfield',
'#title' => $this->t('First name'),
'#required' => TRUE,
];
$form['last_name'] = [
'#type' => 'textfield',
'#title' => $this->t('Last name'),
'#required' => TRUE,
];
$form['actions'] = [
'#type' => 'actions',
'submit' => [
'#type' => 'submit',
'#value' => $this->t('Send'),
],
];
return $form;
}
/**
* {@inheritdoc}
*/
public function validateForm(array &$form, FormStateInterface $form_state) {
// No need for now.
}
/**
* {@inheritdoc}
*/
public function submitForm(array &$form, FormStateInterface $form_state) {
$placeholders = [
'%first_name' => $form_state->getValue('first_name'),
'%last_name' => $form_state->getValue('last_name'),
];
$this->messenger()->addStatus($this->t('Thanks for your submission, %first_name %last_name.', $placeholders));
$form_state->setRedirect('<front>');
}
}
The form asks user to enter first and last name and after submitting displays thanks for that :)
Image
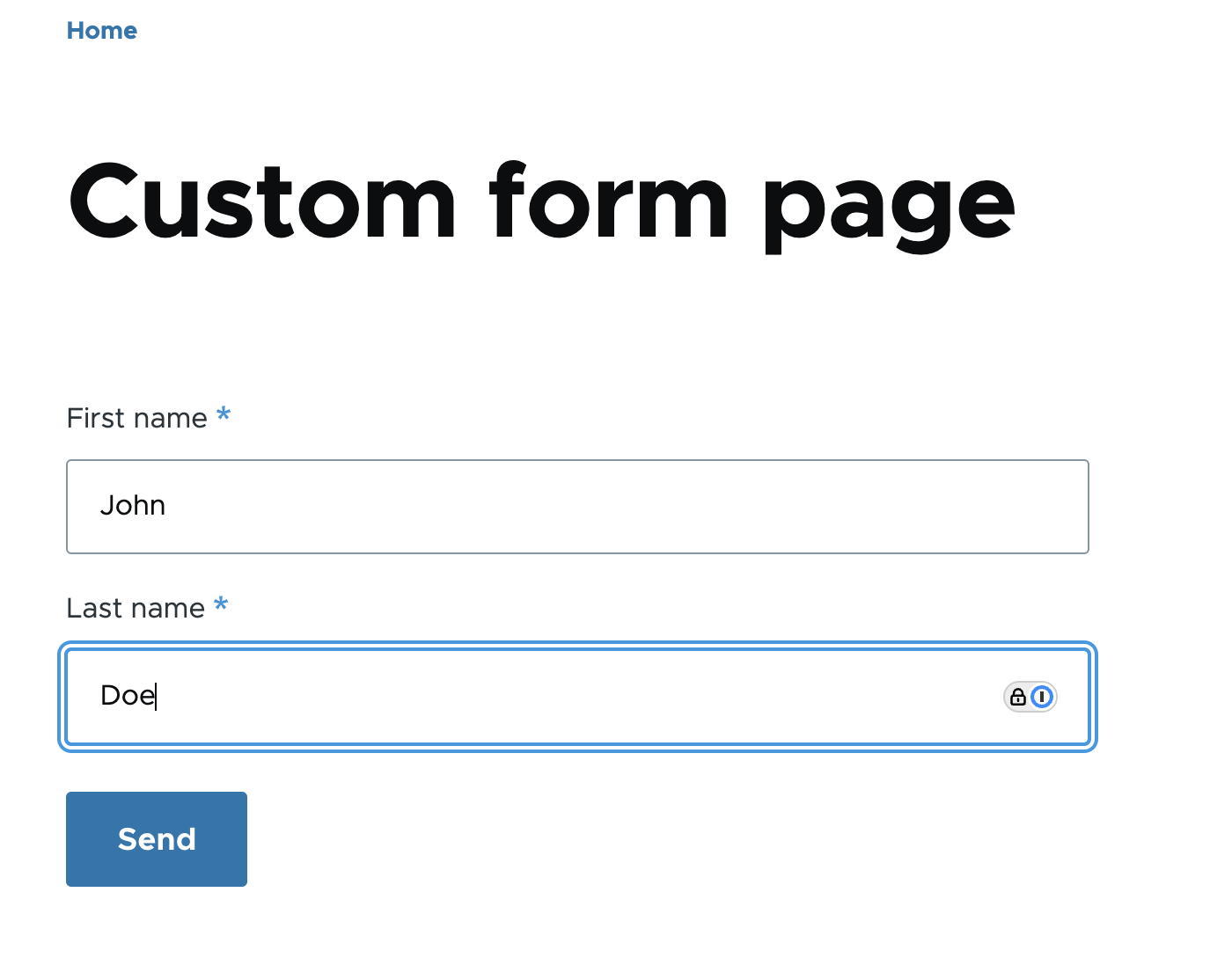
Image
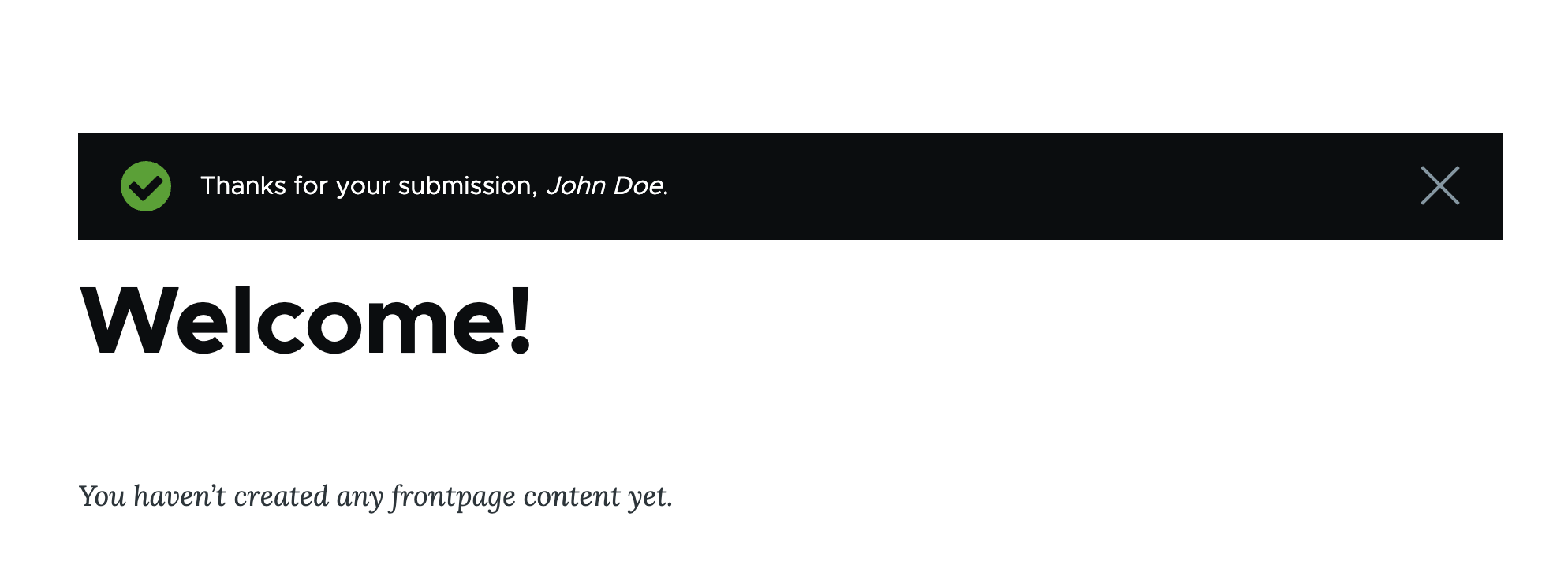